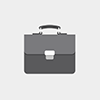
Fundamentals of Java Programming
Overview
The Fundamentals of Java Programming course introduces delegates to programming using the Java language. It explains the concepts of programming using the Java technology stack and gives delegates a practical introduction to the Java landscape.
Prerequisites
Understand the command-line interface, and have used a text editor and a browser. Familiarisation with programming concepts is useful, this knowledge can be obtained by attendance on the Concepts of Programming course.
Objectives
By the end of the course delegates should be able to:
- Understand the Java technology stack
- Understand the Java programming language
- Understand the Java product lifecycle
- Understand object-oriented concepts in Java
- Use the Java language to create Java applications
Outline
Course Contents - DAY 1
Course Introduction
- Administration and Course Materials
- Course Structure and Agenda
- Delegate and Trainer Introductions
Session 1: JAVA TECHNOLOGY PRIMER
- Key Concepts of the Java Language
- Java Technology Landscape
- Java Development Kit
- Java Versions
- Integrated Development Environments (IDE)
Session 2: DEVELOPING AND TESTING A JAVA PROGRAM
- Creating Classes
- Compile and Run Command Line Programs
- Run Applications using the Main Method
- Moving from Procedural to Object Oriented Java
- Compiling and Interpreting Java Code
- Understanding Packages
- Understanding Package-Derived Classes
Session 3: CLASSES, OBJECTS AND RELATIONSHIPS
- Understanding Object Oriented Programming
- Classes and Objects
- Class Compositions and Association
- Class Relationships
- Inner Classes
Course Contents - DAY 2
Session 4: PROGRAMMING WITH JAVA STATEMENTS
- Understanding Primitives
- Declare, Initialise and Utilise Variables and Constants
- Define Variable Scope
- Casting Primitive Types
- Using Operators
Session 5: CONDITIONAL AND LOOP CONSTRUCTS
- Conditional Statements
- Create and Use While Loops
- Create and Use For Loops Including For/Each Syntax
- Create and Use Do/While Loops
- Algorithms and Pseudo-Code
Session 6: CREATE AND USE OBJECTS
- Declare, Instantiate, and Initialize Object Variables
- Understand Object Lifecycle
- Garbage Collection
- Accessing Fields
- Strong Typing
Session 7: UNDERSTANDING METHODS
- Declare and Invoke Methods
- Methods with Return Types
- Static keyword
- Overloading Methods
- Overriding Methods
- Method Outputs
Course Contents - DAY 3
Session 8: ENCAPSULATION AND CONSTRUCTORS
- Data Protection Through Encapsulation
- Access Modifiers
- Object Initialisation with Constructors
- Overloading Constructors
- Default Constructors
Session 9: STRINGS, WRAPPER CLASSES AND CASTING OBJECTS
- String Objects and Methods
- The StringBuilder Class
- Manipulating Strings
- Primitive Wrapper Classes
- Testing equality between objects
- Type Casting and Promotion
- Calendar data
Course Contents - DAY 4
Session 10: COLLECTIONS
- Building Arrays
- Setting Array Values and Looping Through Arrays
- Array Methods and Properties
- Understanding Java Enumerations
- Using the ArrayList Class
Session 11: UNDERSTANDING INHERITANCE
- Inheritance and Class Type
- Overriding Methods
- Abstract Classes
- Interfaces
- Examples of Inheritance and Encapsulation
Session 12: UNDERSTANDING POLYMORPHISM
- Polymorphism Explained
- Inheritance and Interface Polymorphism
- Coding to the Interface
- Examples of Polymorphism
- Simple Lambda expressions
Session 13: HANDLING EXCEPTIONS
- Errors types in Java
- Structured Exception Handling
- Checked Exceptions
- Runtime Exceptions
- Extending the Exception Class
Course Contents - DAY 5
Session 14: UML TO REPRESENT OBJECT-ORIENTED CONCEPTS
- Class, Abstract Class and Interface Diagrams
- Attributes and Operations
- Create Basic UML Diagrams
- Recognise UML Associations
Session 15: JAVA PLATFORMS AND INTEGRATION TECHNOLOGIES
- Multiple Tiers for Java: JSE, JME, JEE
- High Level Overview of Java Remote Method Invocation (RMI)
- High Level Overview of Java Database Connectivity (JDBC)
- Understand the Use of Java Integration API's
Session 16: UNDERSTANDING JAVA CLIENT-SIDE TECHNOLOGIES
- Thin Clients Technologies (HTML and JavaScript)
- JME MIDlets
- Java Applets
- Java Swing
- JavaFX
Session 17: UNDERSTANDING JAVA SERVER-SIDE TECHNOLOGIES
- JEE Tiers and Technologies
- Server Side Components and Options
- Java Dynamic Web Components
- Business Tier Components