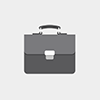
Java Programming
Overview
This Java Programming course focuses on the fundamentals of the Java language, object oriented development and the structure of Java applications. It covers the Java Platform up to version 8.
This hands-on course shows delegates how to develop Java systems that use various core API packages. They will develop programs that support multi-threading and networking. Java has always been associated with GUI development and this course allows delegates to develop graphical applications using JafaFX. They also learn how to access databases and deploy applications.
Prerequisites
Delegates should have experience with another programming language or have attended the pre-requisite course.
Objectives
By the end of the course delegates should be able to:
- Explain the Java architecture
- List and describe the standard Java packages in various versions
- Choose between various design strategies
- Understand the benefits of design patterns
- Create, build, and debug Java projects
- Build and release Java applications
- Store objects using Java's Collection framework
- Write robust applications using Exception handling
- Perform a variety of I/O using Stream and File classes
- Format strings, dates and numbers
- Use Java localisation features
- Develop GUI systems using JavaFX classes
- Raise and respond to events
- Create Java programs with concurrent threads
- Create Network aware applications
- Access databases using JDBC
Outline
Course Introduction
- Administration and Course Materials
- Course Structure and Agenda
- Delegate and Trainer Introductions
Session 1: INTRODUCING JAVA
- The Java Development Environment
- The Java Runtime Environment
- Licensing and OpenJDK
- Compiling and Executing Java Programs
- Java Program types
- Java SE and Java EE
- Integrated Development Environments
Session 2: THE JAVA LANGUAGE
- Variables
- Data Types
- Primitive and Reference Variables
- Declaring, Initializing and Releasing Variables
- Arithmetic and Comparison Operators
- Shortcut Operators
- Short-Circuit Operators
- Shift Operators
- Converting Data Types
- Statements and Semi-Colons
- IF ... ELSE and SWITCH CASE statements
- Loops
- Comments
- Arrays
Session 3: OBJECTS AND CLASSES
- Objects
- Encapsulation
- Classes
- Methods
- Strings
- Packages
- Class Member Data
- Defining and Using Constructors
- Static Initializers
- Static Imports
- Garbage Collection
- Object Lifetime
- Wrapper Classes
- Enumerations
- Inner Classes, Named, Static and Anonymous
Session 4: INHERITANCE
- Classes and Inheritance
- Methods in the Sub Class
- Constructors
- Polymorphism
- Final Classes
- Protected Modifier
- Super keyword and Object class
- Converting Reference Types
Session 5: ABSTRACT CLASSES AND INTERFACES
- Abstraction
- Abstract Classes
- Abstract Class References
- Abstract Methods
- Abstraction Rules and Guidelines
- Interfaces
- Defining and Implementing an Interface
- Type Conversions and Interfaces
- Lambda Expressions
Session 6: DESIGN CONSIDERATIONS
- Composition
- Method Delegation
- Introduction to Design Patterns
- Pattern Examples - Delegate, Singleton, DAO and Decorator
Session 7: GENERICS AND ANNOTATIONS
- User defined Annotations
- Built in Annotations
- Generics
- The Enhanced FOR Loop
- Variable Method Arguments
- Auto Boxing **
Session 8: COLLECTIONS
**
- The java.util and java.util.stream Packages
- Iterator
- Lists
- Maps
- Sets
- Stack and Deque
- Diamond Syntax (Type Inference)
- How to Choose a Collection Class
Session 9: EXCEPTIONS AND ASSERTIONS
- Syntax Errors
- Runtime Errors
- Logical Errors
- Exception Handling
- Exception Objects
- Handling Exceptions
- Try-with-resources
- Multi-catch
- User-Defined Exception Classes
- Exception Handling Guidelines
- Assertions
Session 10: FORMATTING STRINGS, DATES AND NUMBERS
- String Immutability
- String Class Methods
- The StringBuffer and StringBuilder Classes
- Text Input and Parsing
- Text Formatting
- Using Regular Expressions
- Date Formatting
- Calendar and java.time package
- Number Formatting
- Localization
- Resource Bundles
Session 11: CREATING GUIS WITH JAVAFX
- JAVAFX Overview
- Create a JAVAFX GUI
- Event handling
- Using Scene Builder
- Visual effects
- Model View Controller (MVC) Design Pattern
Session 12: FILE AND NETWORK INPUT/OUTPUT
- Byte Oriented Input and Output Streams
- Decoration
- Character Oriented Streams
- Standard I/O Streams
- Examples Using I/O Streams
- Java and URLs
- Handling Files and Directories
- The Path Interface
- Files and Paths class and java.nio.file package
- Reading and Editing Files
- Monitoring with WatchService
Session 13: OBJECT SERIALIZATION
- Introduction
- Examples
- Serialization Issues
- The Externalizable Interface
- Version Serialized Files
Session 14: THREADS AND CONCURRENCY
- What are Threads?
- Creating Threads
- Implementing the Runnable Interface
- Synchronizing Access to Resources
- Communicating Between Threads
- Immutable Objects
- Using the java.util.concurrency Package
- Executors and ThreadPools
Session 15: DATABASE CONNECTION WITH JDBC
- The JDBC API
- Adding JDBC Libraries
- Registering the JDBC Driver
- Connecting to the Database
- Creating and Executing Statements
- The PreparedStatement and CallableStatement Classes
- Using RowSet Classes
- Implementing the Data Access Object Pattern
Session 16: DEPLOYMENT WITH JAR, EAR AND WAR FILES
- Creating Jar Files
- The Jar Manifest
- WAR Files
- EAR Files