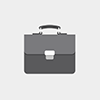
JavaScript Web Development
Overview
The JavaScript Web Development course provides a core understanding of the JavaScript programming language and supporting libraries.
In a practical, hands-on environment, delegates work with JavaScript in a browser environment to compose and manipulate web aspects and components using a variety of techniques.
Prerequisites
An understanding of web markup languages (HTML, XHTML) is a requirement, as JavaScript will be presented in conjunction with web markup and interact with page elements.
Programming experience would be advantageous. While not a specific requirement, it would be beneficial if delegates had some exposure to either a procedural or object oriented language and have sufficient core understanding of programming structures and concepts.
Objectives
Delegates will gather a core understanding of the practical application of the JavaScript language and common libraries in a web context. The core of the language is the main concern, introducing the major features of web programming such as the DOM, browsers, user interaction and working in a browser environment in order to provide a grounding in the major aspects of JavaScript web development.
Outline
Course Contents - DAY 1
Course Introduction
- Administration and Course Materials
- Course Structure and Agenda
- Delegate and Trainer Introductions
Session 1: INTRODUCTION TO JAVASCRIPT WEB DEVELOPMENT
- JavaScript vs. Java
- JavaScript Tasks
- JavaScript Evolution
- Security Overview
- JavaScript Versions and Browser Support
- JavaScript Engines
Session 2: LANGUAGE STRUCTURE
- Character Set
- Case Sensitivity
- Layout and Whitespace
- JavaScript Literals
- Identifiers
- Reserved Words
- Operator Precedence
Session 3: DATATYPES AND VALUES
- Numbers
- Strings
- String Methods
- Booleans
- JavaScript Functions
- The this Keyword
- Objects Introduction
- Arrays Introduction
- Type Conversion
- Primitive Datatype Wrappers
Session 4: VARIABLES, OPERATORS AND EXPRESSIONS
- JavaScript Variables
- JavaScript Expressions
- Arithmetic Operators
- Relational Operators
- String Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- The global Object
Course Contents - DAY 2
Session 5: JAVASCRIPT CONTROL STRUCTURES
- If/Else
- For/For In
- While
- Switch
- Break and Continue
- Using var
- Function
- Return
- JavaScript Exception Handling
- With and Empty Keywords
Session 6: OBJECTS AND ARRAYS
- Object
- Common Properties and Methods
- Dates, Times and Timers
- Classes and Prototyping
- Arrays
- Array Methods
Session 7: JAVASCRIPT FUNCTIONS
- Defining Functions
- Invoking Functions
- Functions as Data
- Anonymous Functions
- Passing Arguments to Functions
Session 8: INTRODUCTION TO REGULAR EXPRESSIONS
- The RegEx Object
- Methods and Usage
- Patterns Matching Examples
- Regular Expressions Tools
Course Contents - DAY 3
Session 9: BROWSER-BASED JAVASCRIPT
- The Window Object
- JavaScript Event Handling
- JavaScript Form Handling
- The Document Object Model
- Cookies
- Alternative Storage Options
Session 10: JAVASCRIPT TOOLS
- Profiling JavaScript
- Firebug
- Fiddler
- YSlow
- Other JavaScript Tools
Session 11: JAVASCRIPT EXTRAS
- Direct Web Remoting (DWR)
- Node Discussion
- AHAH/JAH
- AJAX
- Working with Structured Data
- JavaScript Libraries/Frameworks
Session 12:USING JQUERY
- The jQuery Library
- jQuery Core
- jQuery Effects and Events
- jQuery Ajax